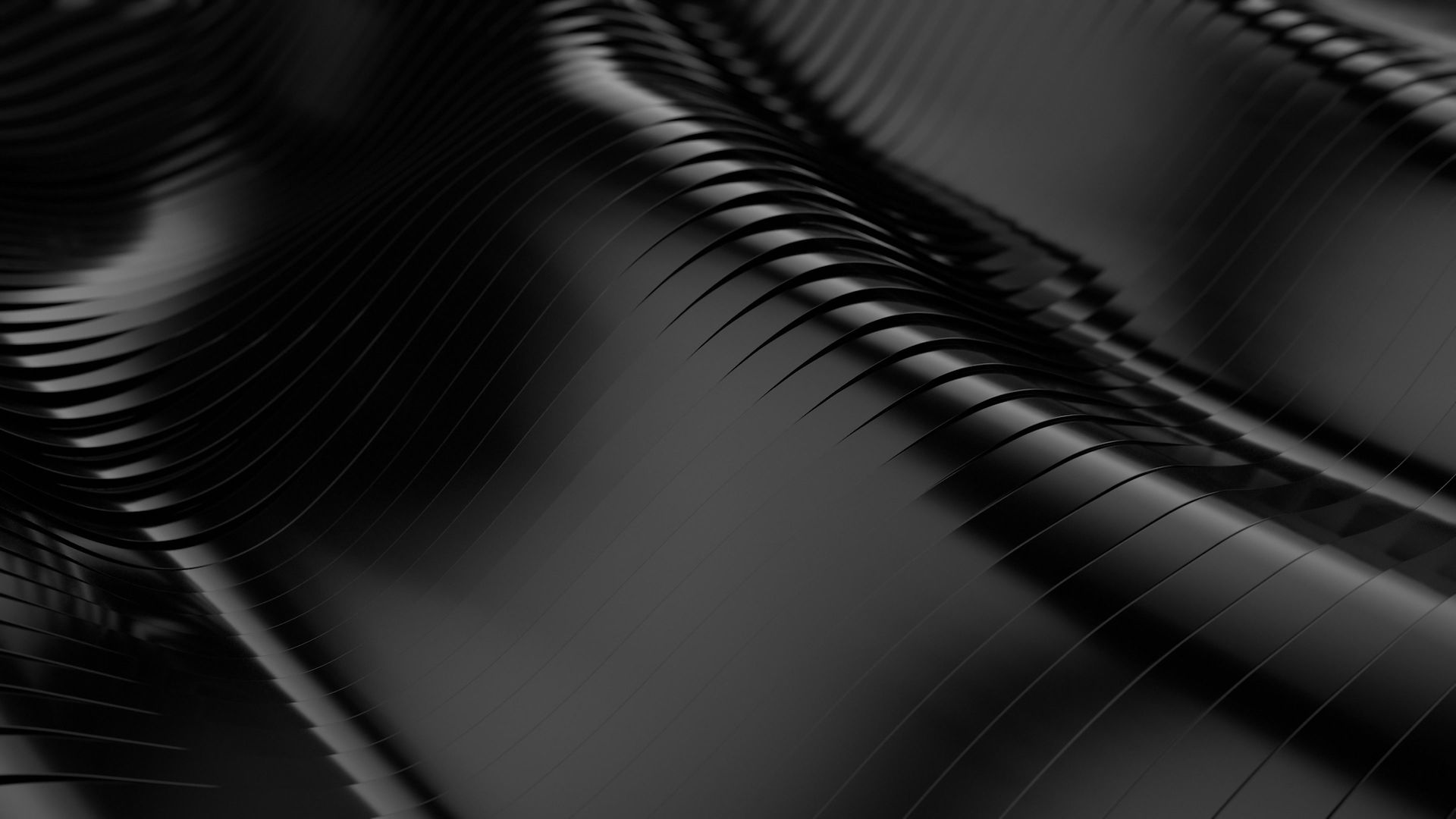
Canny Edge Detection
Summary
Canny Edge Detection with open cv example.
Detect object's edges in the image.
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread(path)
img = cv2.cvtColor(img,cv2.COLOR_BGR2RGB)
plt.figure(1)
plt.imshow(img)
canny = cv2.Canny(img,127,127)
plt.figure(2)
plt.imshow(canny,cmap='gray')
## choosing thresholds
med_val = np.median(img)
lower = int(max(0,0.7*med_val))
upper = int(min(255,1.3*med_val))
canny1 = cv2.Canny(img,lower,upper)
plt.figure(3)
plt.imshow(canny1,cmap='gray')
blurred_img = cv2.blur(img,ksize=(5,5))
canny2 = cv2.Canny(blurred_img,lower,upper)
plt.figure(4)
plt.imshow(canny2,cmap='gray')


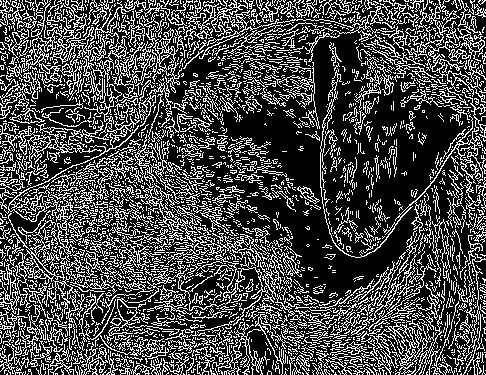
